
Hello everyone, 👋 I'm currently experiencing a problem with my SvelteKit application. :svelte: I have configured my application to handle user sessions using the Appwrite SDK for Node.js because of SSR. When I try to add or delete documents in my collection from my application, I get the following error: "Permissions must be one of: (any, guests)". I set these security rules for my collection because I want only the right user can read, update, delete
User -> read Document security is enabled
If you need more information or a code, don't hesitate to ask me.
Thank you in advance for your help 😄

Here's the code :
lib/server/ideas.ts
export const getIdeas = async (): Promise<Idea[]> => {
try {
const client = new Client().setEndpoint(APPWRITE_ENDPOINT).setProject(APPWRITE_PROJECT);
const databases = new Databases(client);
const ideas = await databases.listDocuments(STARTER_DATABASE_ID, STARTER_COLLECTION_ID);
return ideas.documents as Idea[];
} catch (e) {
...
}
return [];
};
export const addIdea = async (idea: Idea) => {
try {
const client = new Client().setEndpoint(APPWRITE_ENDPOINT).setProject(APPWRITE_PROJECT);
const databases = new Databases(client);
return databases.createDocument(
STARTER_DATABASE_ID,
STARTER_COLLECTION_ID,
idea.$id,
{
userId: idea.userId,
title: idea.title,
description: idea.description
},
[
Permission.read(Role.user(idea.userId)),
Permission.update(Role.user(idea.userId)),
Permission.delete(Role.user(idea.userId))
]
);
} catch (e) {
...
}
};
export const deleteIdea = async (id: string) => {
try {
const client = new Client().setEndpoint(APPWRITE_ENDPOINT).setProject(APPWRITE_PROJECT);
const databases = new Databases(client);
return databases.deleteDocument(STARTER_DATABASE_ID, STARTER_COLLECTION_ID, id);
} catch (e) {
..
}
};
Recommended threads
- document.update
Invalid relationship value! The data I'm passing is not null and all the rest I'm just updating the document and not the relationship
- Cannot create documents with encrypted a...
Hello. I wonder if nobody actually uses these things, however: If you create an string attribute through the API with encrypt: true (why only the api..?), th...
- rate limit exceeded
Hello, Im testing my app and it keeps rate limiting every so often. I see in docs this is per hour, is there anyway to remove or change the rate limit for testi...
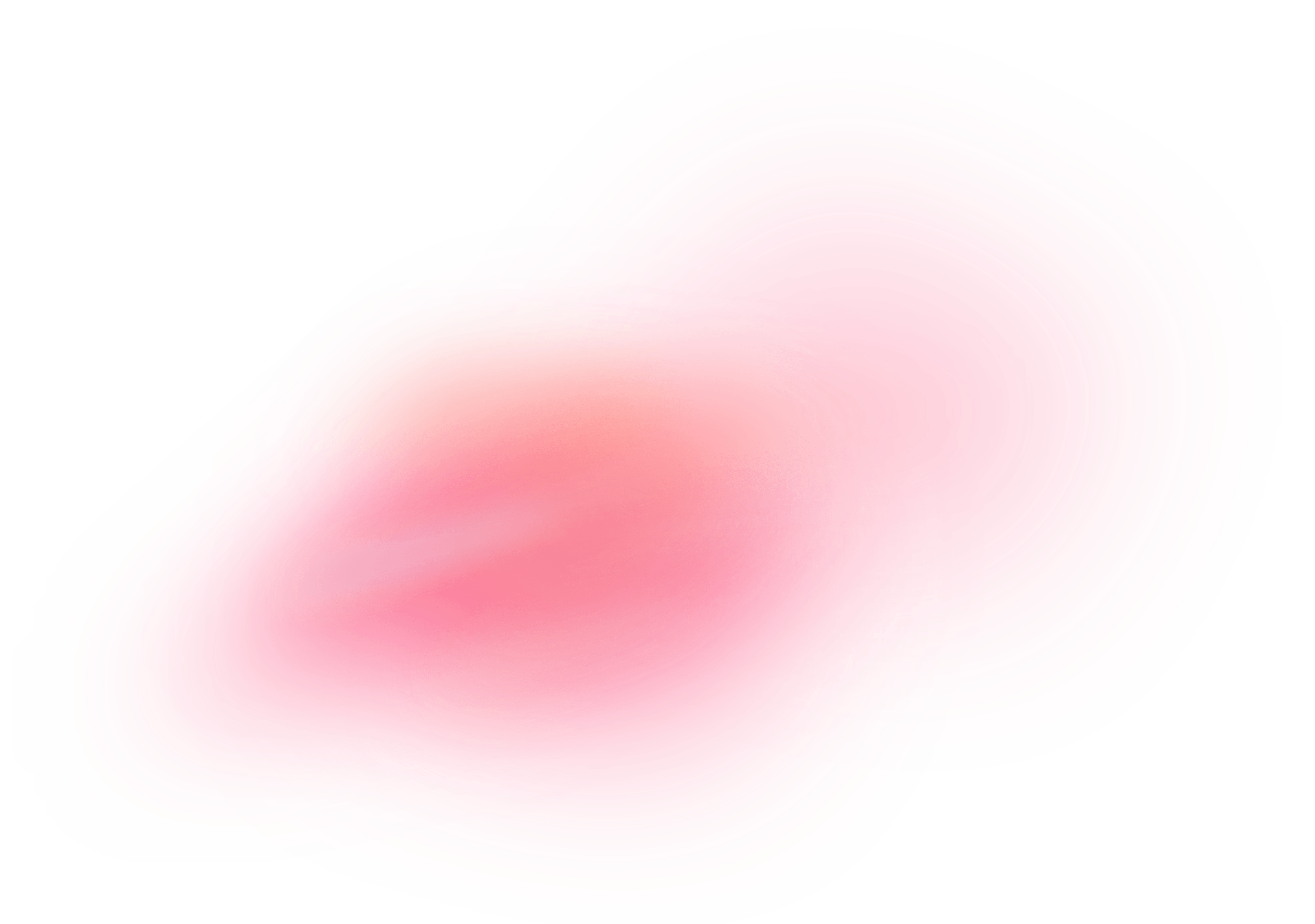