Learn how to setup your first Nuxt project powered by Appwrite.
Create project
Head to the Appwrite Console.
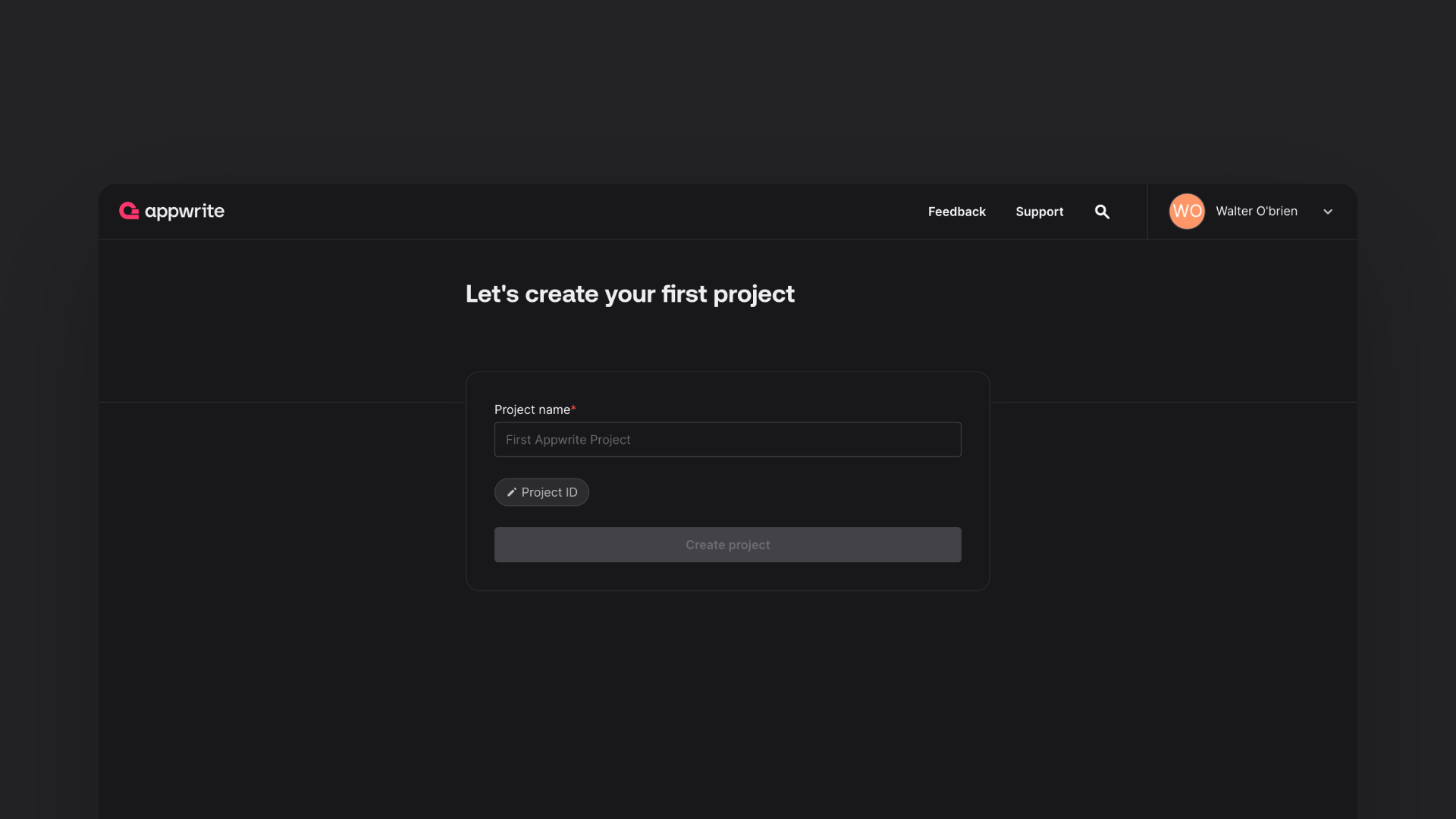
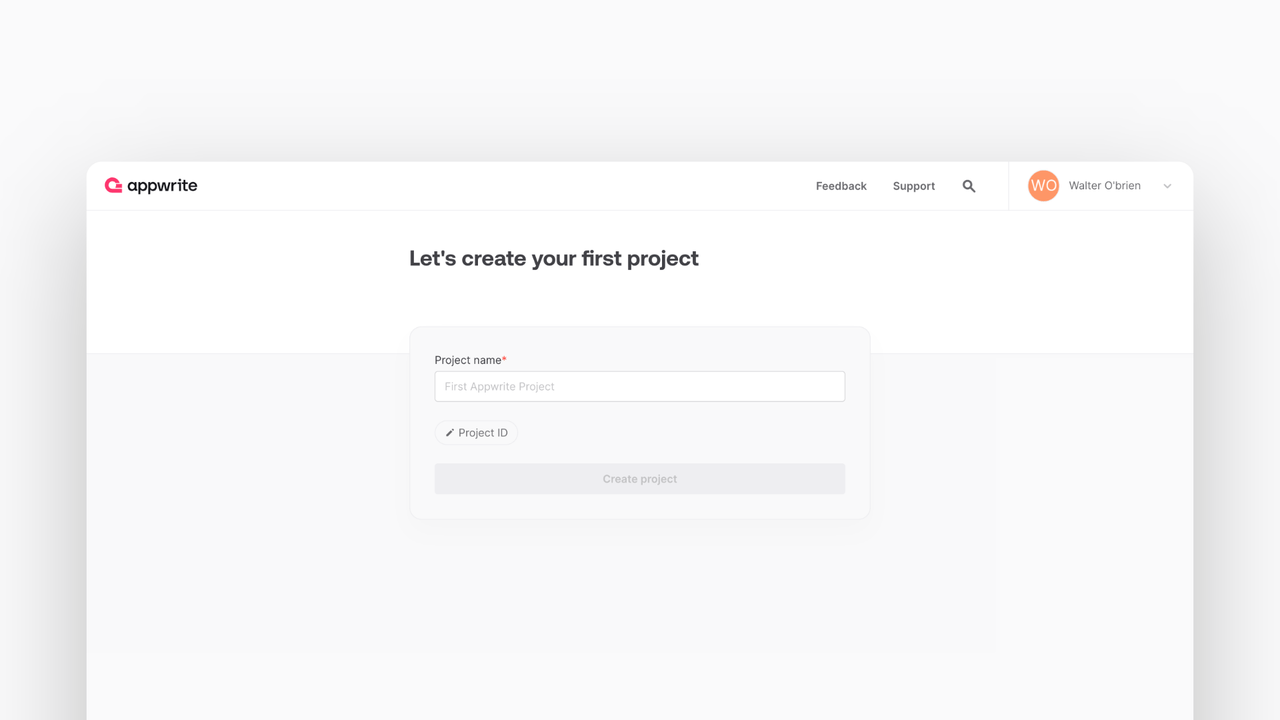
If this is your first time using Appwrite, create an account and create your first project.
Then, under Add a platform, add a Web app. The Hostname should be localhost.
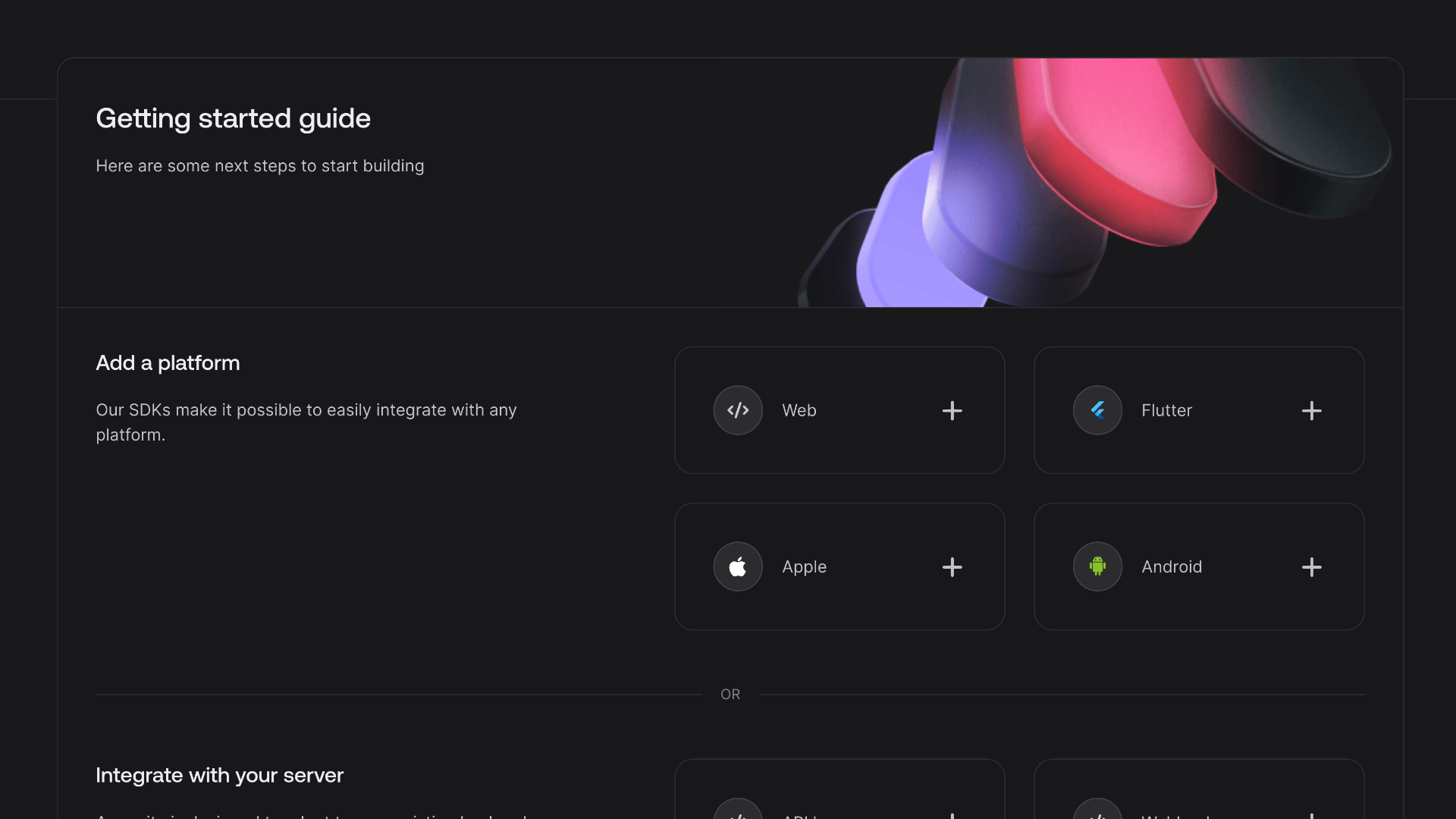
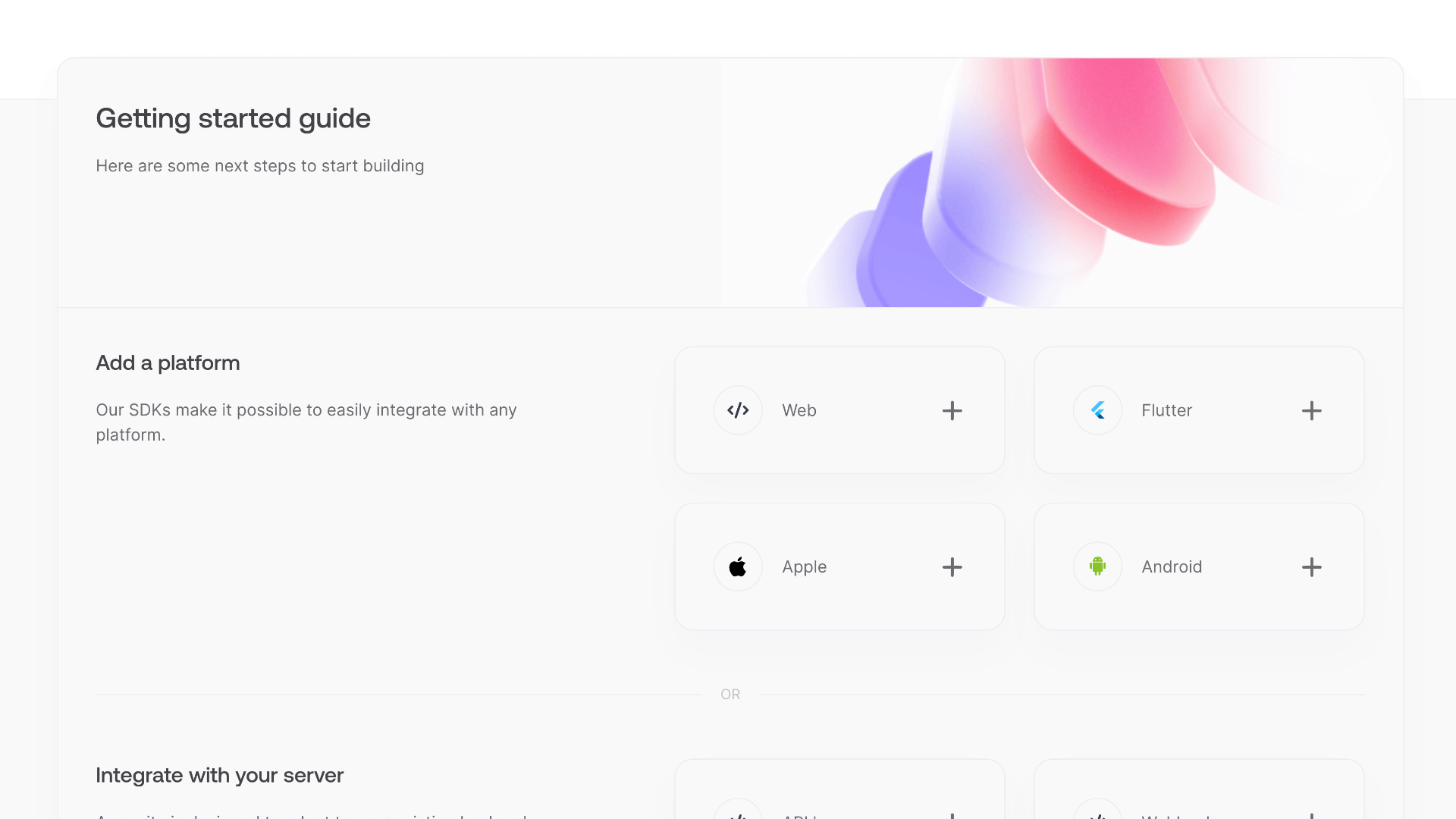
You can skip optional steps.
Create Nuxt project
Create a Nuxt project.
Shell
npx nuxi@latest init my-app && cd my app
Install Appwrite
Install the JavaScript Appwrite SDK.
Shell
npm install appwrite@13.0.2
Import Appwrite
Find your project's ID in the Settings page.
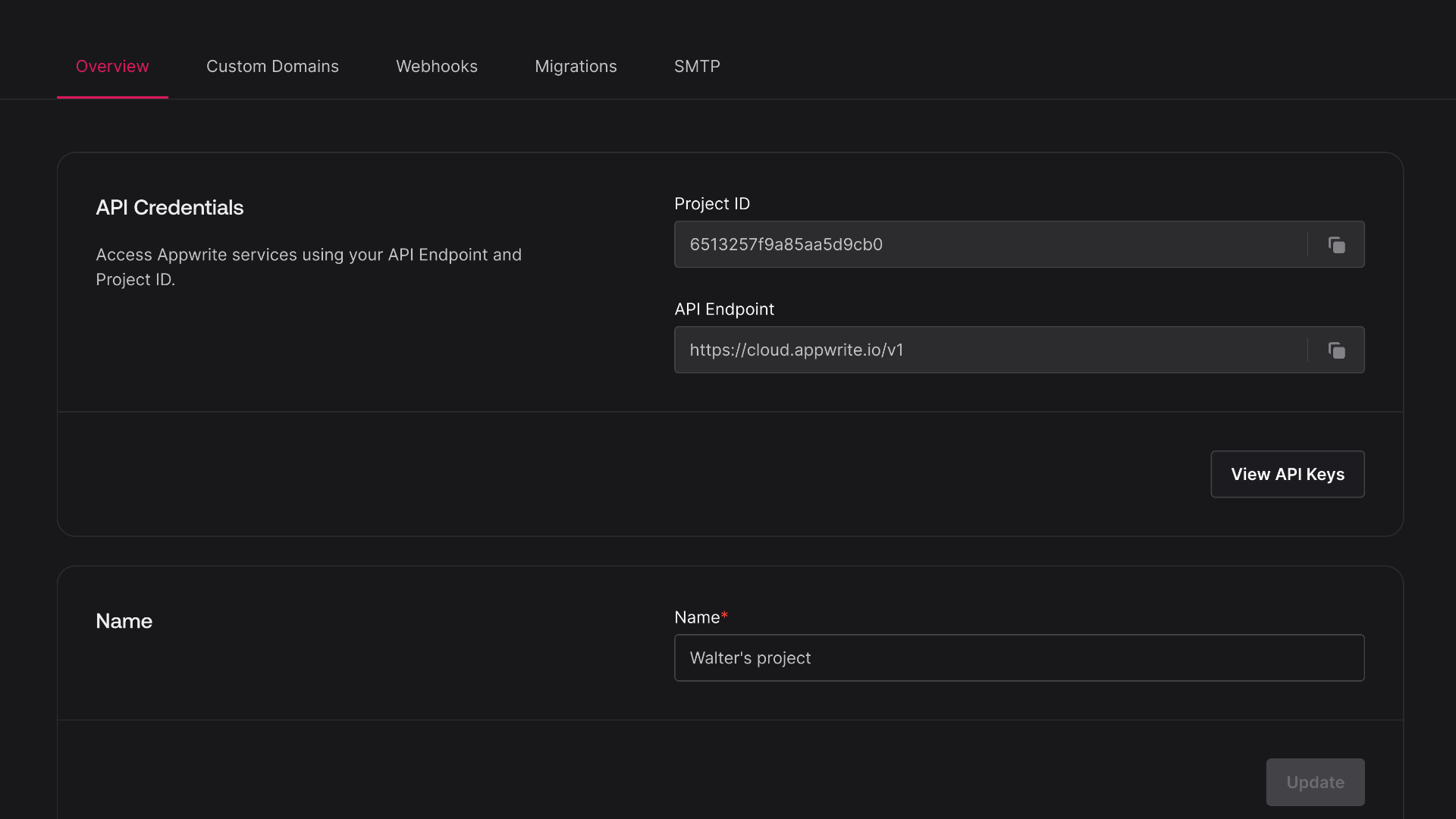
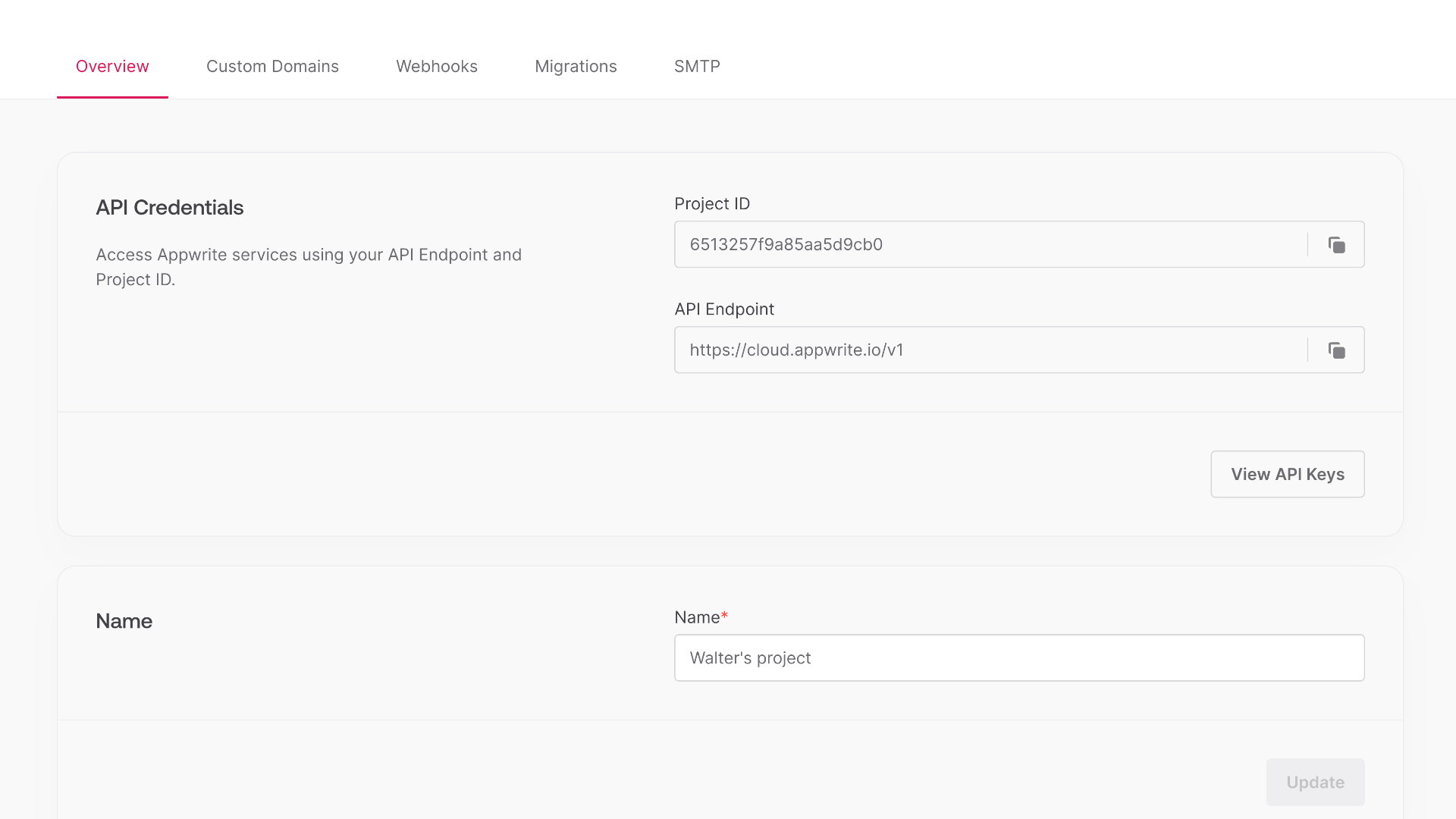
Create a new file utils/appwrite.js and add the following code to it, replace <YOUR_PROJECT_ID> with your project ID.
Web
import { Client, Account} from 'appwrite';
export const client = new Client();
client
.setEndpoint('https://cloud.appwrite.io/v1')
.setProject('<YOUR_PROJECT_ID>'); // Replace with your project ID
export const account = new Account(client);
export { ID } from 'appwrite';
Create a login page
Add the following code to app.vue.
HTML
<script setup>
import { ref } from 'vue';
import { account, ID } from './utils/appwrite.js';
const loggedInUser = ref(null);
const email = ref('');
const password = ref('');
const name = ref('');
const login = async (email, password) => {
await account.createEmailSession(email, password);
loggedInUser.value = await account.get();
};
const register = async () => {
await account.create(ID.unique(), email.value, password.value, name.value);
login(email.value, password.value);
};
const logout = async () => {
await account.deleteSession('current');
loggedInUser.value = null;
};
</script>
<template>
<div>
<p>
{{ loggedInUser ? `Logged in as ${loggedInUser.name}` : 'Not logged in' }}
</p>
<form>
<input type="email" placeholder="Email" v-model="email" />
<input type="password" placeholder="Password" v-model="password" />
<input type="text" placeholder="Name" v-model="name" />
<button type="button" @click="login(email, password)">Login</button>
<button type="button" @click="register">
Register
</button>
<button type="button" @click="logout">
Logout
</button>
</form>
</div>
</template>
All set
Run your project with npm run dev -- --open --port 3000 and open Localhost on Port 3000 in your browser.